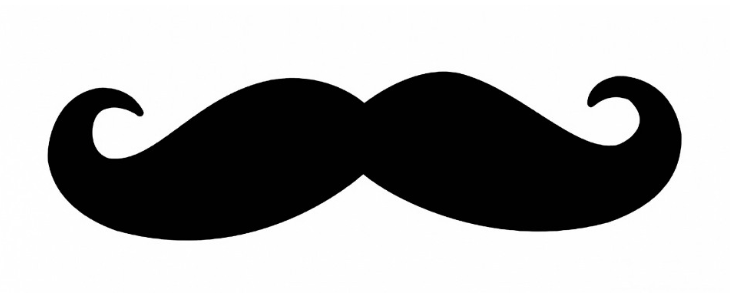
Published 2022. 4. 21. 13:13
2022.04.21 - [무조건 따라하기/java] - 스프링 부트와 AWS로 혼자 구현하는 웹 서비스 - 4장 (1)
스프링 부트와 AWS로 혼자 구현하는 웹 서비스 - 4장 (1)
whitewise95.tistory.com
04 머스테치로 화면 구성하기
4.4 전체 조회 화면 만들기
1. 다음과 같이 하겠습니다
- 전체조회를 위해 index.mustache ui를 변경하겠습니다.
- dto패키지안에 PostsListResponseDto 클래스를 생성합니다.
- PostsRepository, PostsService, IndexController 순으로 코드를 추가하겠습니다.
index.mustache
<h1>스프링부트로 시작하는 웹 서비스 Ver.2</h1>
<div class="col-md-12">
<div class="row">
<div class="col-md-6">
<a href="/posts/save" role="button" class="btn btn-primary">글 등록</a>
</div>
<br>
<!-- 목록 출력 영역 -->
<table class="table table-horizontal table-bordered">
<thead class="thead-strong">
<tr>
<th>게시글번호</th>
<th>제목</th>
<th>작성자</th>
<th>최종수정일</th>
</tr>
</thead>
<tbody id="tbody">
{{#posts}}
<tr>
<td>{{id}}</td>
<td>{{title}}</td>
<td>{{author}}</td>
<td>{{modifiedDate}}</td>
</tr>
{{/posts}}
</tbody>
</table>
</div>
PostsRepository
//SpringDataJpa에서 제공하지 않는 메소드는 이 처럼 @Query 를 사용합니다.
@Query("SELECT p FROM Posts p ORDER BY p.id DESC")
List<Posts> findAllDesc();
PostsListResponseDto
@Getter
public class PostsListResponseDto {
private Long id;
private String title;
private String author;
private LocalDateTime modifiedDate;
public PostsListResponseDto(Posts entity) {
this.id = entity.getId();
this.title = entity.getTitle();
this.author = entity.getAuthor();
this.modifiedDate = entity.getModifiedDate();
}
}
PostsService
@Transactional(readOnly = true)
public List<PostsListResponseDto> findAllDesc() {
return postsRepository.findAllDesc().stream()
.map(PostsListResponseDto::new)
.collect(Collectors.toList());
}
IndexController
package com.jojoldu.book.springboot.web;
import com.jojoldu.book.springboot.service.PostsService;
import lombok.RequiredArgsConstructor;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@RequiredArgsConstructor
@Controller
public class IndexController {
//추가됨
private final PostsService postsService;
// model 과 model.addAttribute 추가됨
@GetMapping("/")
public String index(Model model) {
model.addAttribute("posts", postsService.findAllDesc());
return "index";
}
@GetMapping("/posts/save")
public String postsSave() {
return "posts-save";
}
}
2. 브라우저로 접근을 하고 글을 등록하면 글이 등록된걸 확인 할 수 있습니다.
4.5 게시글 수정, 삭제 화면만들기
- 이미 만들어진 PostsApiController의 update메소드를 이용해 수정기능을 개발합니다.
- posts-update.mustache 생성합니다.
- index.js파일 함수 추가
- index.mustache ui추가
- IndexContorller 메소드 하나를 추가합니다.
posts-update.mustache
{{>layout/header}}
<h1>게시글 수정</h1>
<div class="col-md-12">
<div class="col-md-4">
<form>
<div class="form-group">
<label for="title">글 번호</label>
<input type="text" class="form-control" id="id" value="{{post.id}}" readonly>
</div>
<div class="form-group">
<label for="title">제목</label>
<input type="text" class="form-control" id="title" value="{{post.title}}">
</div>
<div class="form-group">
<label for="author"> 작성자 </label>
<input type="text" class="form-control" id="author" value="{{post.author}}" readonly>
</div>
<div class="form-group">
<label for="content"> 내용 </label>
<textarea class="form-control" id="content">{{post.content}}</textarea>
</div>
</form>
<a href="/" role="button" class="btn btn-secondary">취소</a>
<button type="button" class="btn btn-primary" id="btn-update">수정 완료</button>
<button type="button" class="btn btn-danger" id="btn-delete">삭제</button>
</div>
</div>
{{>layout/footer}}
index.js
var main = {
init : function () {
var _this = this;
$('#btn-save').on('click', function () {
_this.save();
});
$('#btn-update').on('click', function () {
_this.update();
});
},
save : function () {
var data = {
title: $('#title').val(),
author: $('#author').val(),
content: $('#content').val()
};
$.ajax({
type: 'POST',
url: '/api/v1/posts',
dataType: 'json',
contentType:'application/json; charset=utf-8',
data: JSON.stringify(data)
}).done(function() {
alert('글이 등록되었습니다.');
window.location.href = '/';
}).fail(function (error) {
alert(JSON.stringify(error));
});
},
update : function () {
var data = {
title: $('#title').val(),
content: $('#content').val()
};
var id = $('#id').val();
$.ajax({
type: 'PUT',
url: '/api/v1/posts/'+id,
dataType: 'json',
contentType:'application/json; charset=utf-8',
data: JSON.stringify(data)
}).done(function() {
alert('글이 수정되었습니다.');
window.location.href = '/';
}).fail(function (error) {
alert(JSON.stringify(error));
});
},
};
main.init();
index.mustache 의 title 에 <a href="/posts/update/{{id}}"> 를 추가합니다.
{{#posts}}
<tr>
<td>{{id}}</td>
<td><a href="/posts/update/{{id}}">{{title}}</a></td>
<td>{{author}}</td>
<td>{{modifiedDate}}</td>
</tr>
{{/posts}}
IndexContorller
@GetMapping("/posts/update/{id}")
public String postsUpdate(@PathVariable Long id, Model model) {
PostsResponseDto dto = postsService.findById(id);
model.addAttribute("post", dto);
return "posts-update";
}
2. 브라우저로 접근하여 확인합니다.
3. 삭제 이베트도 추가합니다.
- index.js에 함수를 추가합니다.
- PostsService에 메소드를 추가합니다.
- PostsApiController에 메소드를 추가합니다.
- 브라우저로 접근해 삭제를 확인합니다.
index.js
var main = {
init : function () {
var _this = this;
$('#btn-save').on('click', function () {
_this.save();
});
$('#btn-update').on('click', function () {
_this.update();
});
$('#btn-delete').on('click', function () {
_this.delete();
});
},
save : function () {
var data = {
title: $('#title').val(),
author: $('#author').val(),
content: $('#content').val()
};
$.ajax({
type: 'POST',
url: '/api/v1/posts',
dataType: 'json',
contentType:'application/json; charset=utf-8',
data: JSON.stringify(data)
}).done(function() {
alert('글이 등록되었습니다.');
window.location.href = '/';
}).fail(function (error) {
alert(JSON.stringify(error));
});
},
update : function () {
var data = {
title: $('#title').val(),
content: $('#content').val()
};
var id = $('#id').val();
$.ajax({
type: 'PUT',
url: '/api/v1/posts/'+id,
dataType: 'json',
contentType:'application/json; charset=utf-8',
data: JSON.stringify(data)
}).done(function() {
alert('글이 수정되었습니다.');
window.location.href = '/';
}).fail(function (error) {
alert(JSON.stringify(error));
});
},
delete : function () {
var id = $('#id').val();
$.ajax({
type: 'DELETE',
url: '/api/v1/posts/'+id,
dataType: 'json',
contentType:'application/json; charset=utf-8'
}).done(function() {
alert('글이 삭제되었습니다.');
window.location.href = '/';
}).fail(function (error) {
alert(JSON.stringify(error));
});
}
};
main.init();
PostsService
@Transactional
public void delete (Long id) {
Posts posts = postsRepository.findById(id)
.orElseThrow(() -> new IllegalArgumentException("해당 사용자가 없습니다. id=" + id));
postsRepository.delete(posts);
}
PostsApiController
@DeleteMapping("/api/v1/posts/{id}")
public Long delete(@PathVariable Long id) {
postsService.delete(id);
return id;
}
브라우저로 접근해 삭제를 확인합니다.
'무조건 따라하기 > Spring Boot 기반 Web Service' 카테고리의 다른 글
스프링부트와 AWS로 혼자 구현하는 웹 서비스 - 05장 (2) 구글로그인 - 구글크라우드 설정 (0) | 2022.04.29 |
---|---|
스프링부트와 AWS로 혼자 구현하는 웹 서비스 - 05장 (1) 스프링부트 1.5 vs 스프링부트 2.0 (0) | 2022.04.29 |
스프링 부트와 AWS로 혼자 구현하는 웹 서비스 - 4장 (1) (0) | 2022.04.21 |
스프링 부트와 AWS로 혼자 구현하는 웹 서비스 - 3장 (2) (0) | 2022.04.20 |
스프링 부트와 AWS로 혼자 구현하는 웹 서비스 - 3장 (1) (0) | 2022.04.20 |