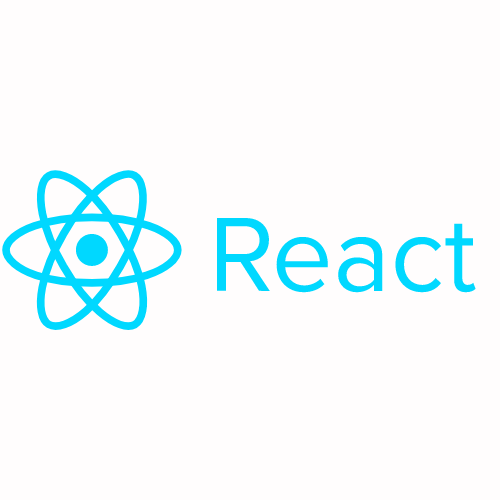
폴더구조
src/redux/config/configStore.js 생성
import {createStore} from "redux";
import {combineReducers} from "redux";
const rootReducer = combineReducers({
// modules
});
const store = createStore(rootReducer);
export default store;
src/redux/modules/counter.js 생성
const initialState = {
number: 0
}
const counter = (state = initialState, action) => {
switch(action.type) {
// type에 맞는 로직 생성
default:
return state;
}
}
export default counter;
configStore.js 로직 추가
import {createStore} from "redux";
import {combineReducers} from "redux";
import counter from "../modules/counter"; //추가한 로직
const rootReducer = combineReducers({
counter, //추가한 로직
});
const store = createStore(rootReducer);
export default store;
리덕스 사용
index.js 로직 수정
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import { Provider } from 'react-redux';
import store from './redux/config/configStore';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<Provider store={store}>
<App />
</Provider>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Redux useSelecter 및 dispatch
- View 에서 액션이 일어난다.
- dispatch 에서 action이 일어나게 된다.
- action에 의한 reducer 함수가 실행되기 전에 middleware가 작동한다.
- middleware 에서 명령내린 일을 수행하고 난뒤, reducer 함수를 실행한다. (3, 4번은 아직 몰라도 됩니다!)
- reducer 의 실행결과 store에 새로운 값을 저장한다.
- store의 state에 subscribe 하고 있던 UI에 변경된 값을 준다. 출처 : https://velog.io/@annahyr/리덕스-흐름-이해하기
useSelecter
Redux는 useSelecter로 counter를 조회할 수 있다.
// src/App.js
import React from "react";
import { useSelector } from "react-redux"; // import 해주세요.
const App = () => {
const counter = useSelector((state) => state.counter); // 추가해주세요.
console.log(counter);
return <div></div>;
}
export default App;
dispatch
useDispatch(); 함수를 사용해서 dispatch를 사용할 수 있다.
예시처럼 디스패치를 이용해서 액션객체를 리듀서로 보낼 수 있습니다.
import React from "react";
import { useDispatch } from "react-redux"; // import 해주세요.
const App = () => {
const dispatch = useDispatch(); // dispatch 생성
return (
<div>
<button
// 이벤트 핸들러 추가
onClick={() => {
// 마우스를 클릭했을 때 dispatch가 실행되고, ()안에 있는 액션객체가 리듀서로 전달된다.
dispatch({ type: "PLUS_ONE" });
}}
>
+ 1
</button>
</div>
);
};
export default App;
리듀서에서 type이 PLUS_ONE 일 경우로 핸들링해준다.
// src/modules/counter.js
// 초기 상태값
const initialState = {
number: 0,
};
// 리듀서
const counter = (state = initialState, action) => {
console.log(action);
switch (action.type) {
// PLUS_ONE이라는 case를 추가한다.
// 여기서 말하는 case란, action.type을 의미한다.
// dispatch로부터 전달받은 action의 type이 "PLUS_ONE" 일 때
// 아래 return 절이 실행된다.
case "PLUS_ONE":
return {
// 기존 state에 있던 number에 +1을 더한다.
number: state.number + 1,
};
default:
return state;
}
};
// 모듈파일에서는 리듀서를 export default 한다.
export default counter;
'몰아 넣기' 카테고리의 다른 글
[리액트] useEffect란? 그리고 의존성배열 (0) | 2024.04.16 |
---|---|
[CSS] 자주쓰는 CSS (0) | 2024.04.15 |
[리액트] 앱생성 및 리액트 자주쓰는 패키지 정리.ver1 (0) | 2024.03.11 |
[예제] Java PDF to JPG 및 pdf (0) | 2024.01.15 |
[JAVA] JavaIo OutPutStream에 대해서 (1) | 2024.01.11 |